
1. 문제
TapeEquilibrium coding task - Learn to Code - Codility
Minimize the value |(A[0] + ... + A[P-1]) - (A[P] + ... + A[N-1])|.
app.codility.com
A non-empty array A consisting of N integers is given. Array A represents numbers on a tape.
Any integer P, such that 0 < P < N, splits this tape into two non-empty parts: A[0], A[1], ..., A[P − 1] and A[P], A[P + 1], ..., A[N − 1].
The difference between the two parts is the value of: |(A[0] + A[1] + ... + A[P − 1]) − (A[P] + A[P + 1] + ... + A[N − 1])|
In other words, it is the absolute difference between the sum of the first part and the sum of the second part.
For example, consider array A such that:
A[0] = 3
A[1] = 1
A[2] = 2
A[3] = 4
A[4] = 3
We can split this tape in four places:
P = 1, difference = |3 − 10| = 7
P = 2, difference = |4 − 9| = 5
P = 3, difference = |6 − 7| = 1
P = 4, difference = |10 − 3| = 7
Write a function:
class Solution { public int solution(int[] A); }
that, given a non-empty array A of N integers, returns the minimal difference that can be achieved.
For example, given:
A[0] = 3
A[1] = 1
A[2] = 2
A[3] = 4
A[4] = 3
the function should return 1, as explained above.
Write an efficient algorithm for the following assumptions:
N is an integer within the range [2..100,000];
each element of array A is an integer within the range [−1,000..1,000].
2. 풀이
1. A 배열을 누적합으로 변경한다. A[i]는 i번째까지의 누적합이다.
2. answer을 먼저 최댓값으로 초기화한다.
3. 0번부터 len-1까지 탐색하면서 최솟값 answer을 구한다. P가 i+1일 때, P-1까지의 합인 A[i]와 그 나머지(A[len-1]-A[i])의 차이를 계산하면 된다. len-1까지 탐색하는 이유는 P<N이기 때문이다. P-1 = i < N - 1이어야 한다.
public class TapeEquilibrium {
public int solution(int[] A) {
int len = A.length;
for(int i=1;i<len;i++) A[i] += A[i-1];
int answer = Integer.MAX_VALUE;
for(int i=0;i<len-1;i++){
answer = Math.min(answer, Math.abs(2*A[i]-A[len-1]));
}
return answer;
}
}
3. 결과

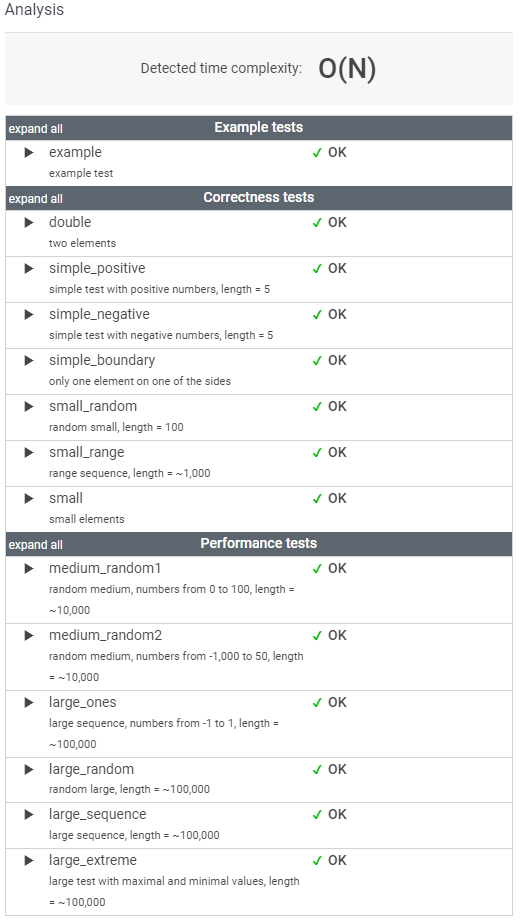
'코딩테스트 > ELSE' 카테고리의 다른 글
[Codility] OddOccurrencesInArray - JAVA (0) | 2023.06.22 |
---|---|
[Codility] CyclicRotation - Java (0) | 2023.06.22 |
[Softeer] 전광판 - JAVA (1) | 2023.05.14 |
[COS PRO 기출문제] 소용돌이 수 - JAVA (1) | 2023.05.11 |
[Softeer] 장애물 인식 프로그램 - JAVA (0) | 2023.05.10 |
댓글